Introduction to JavaScript’s Not Equal and Comparison Operators
JavaScript’s inequality operator, commonly symbolized as !=
, plays a pivotal role in decision-making processes within code. It is used to compare two values, determining whether they are not equal to each other. For instance, if x != y
, the result is true if x
and y
are not the same. It’s a fundamental aspect of JavaScript that allows for dynamic and conditional programming. This section will introduce this operator and its significance in various coding scenarios.
The Role and Importance of Comparison Operators in JavaScript
Understanding the “!=” Operator in JavaScript
The “!=” operator is a cornerstone in JavaScript’s suite of comparison tools. It’s used to test the inequality of two expressions. For example, consider the code snippet:
if (4 != 5) {
console.log("4 is not equal to 5");
}
This code will output "4 is not equal to 5"
because 4 and 5 are indeed not equal. This operator is crucial for controlling the flow of the program based on different conditions.
Practical Implementation and Examples of “!=”
Implementing the !=
operator is straightforward yet powerful. Let’s take another example:
let a = 10;
let b = "10";
if (a != b) {
console.log("a and b are not equal");
} else {
console.log("a and b are equal");
}
Even though a
and b
have the same value, they are of different types (number and string), yet this code will output "a and b are equal"
because the !=
operator does not check for type equality. This demonstrates the subtlety in using !=
effectively.
Investigating Other Comparison Operators in JavaScript
Apart from !=
, JavaScript includes a variety of other comparison operators like ==
, ===
, >
, <
, >=
, and <=
. Each of these operators serves a specific purpose in comparing values. For instance, ===
checks for both value and type equality, making it a stricter form of comparison than ==
. Understanding the appropriate context for each operator is key to effective coding.
Demonstrative Examples and Their Analysis
Let’s explore more examples:
let x = 3;
let y = 5;
console.log(x > y); // false
console.log(x < y); // true
console.log(x >= 3); // true
console.log(x <= 2); // false
These snippets demonstrate the use of greater than, less than, greater than or equal to, and less than or equal to operators, respectively. Each operator serves a specific role in comparing values and drives the logical flow of the program.
Concluding Thoughts on JavaScript’s Inequality Operator
To conclude, the !=
operator, along with its counterparts, forms the backbone of logical decision-making in JavaScript. It’s crucial for developers to understand the nuances of these operators to write efficient and accurate JavaScript code. While !=
checks for inequality, understanding its behavior concerning data types and how it differs from the strict inequality operator !==
is vital for any JavaScript programmer.
Comprehensive Exploration of JavaScript Comparison and Logical Operators
Delving into Comparison Operators in JavaScript
JavaScript’s comparison operators are essential tools for decision-making in code. They allow you to compare two values, determining whether one is greater than, less than, equal to, or not equal to another. Understanding these operators is crucial for control flow in applications.
Practical Uses of Comparison Operators
Comparison operators are widely used in conditional statements like if
, while
, and for
loops. For example, in an if
statement, you might check if a user’s age is greater than 18 to determine eligibility:
let userAge = 19;
if (userAge > 18) {
console.log("User is eligible.");
}
Case Studies and Examples
In a shopping cart application, comparison operators can be used to check if the total amount exceeds a certain limit for a discount:
let cartTotal = 250;
if (cartTotal >= 200) {
console.log("Eligible for discount.");
}
Examination of JavaScript’s Logical Operators
Logical operators like &&
(AND), ||
(OR), and !
(NOT) are used to combine multiple conditions. They are pivotal in constructing complex logical expressions.
Introduction to the Conditional (Ternary) Operator
The ternary operator is a shorthand for the if-else
statement. It takes three operands and is used for assigning a value based on a condition. The syntax is condition ? exprIfTrue : exprIfFalse
.
Syntax Breakdown
Here’s an example of the ternary operator:
let age = 20;
let beverage = age >= 18 ? "Beer" : "Juice";
console.log(beverage); // "Beer"
Demonstrative Example of the Ternary Operator
In a web application, the ternary operator can be used to quickly decide which message to display to the user:
let loggedIn = true;
let message = loggedIn ? "Welcome back!" : "Please log in.";
console.log(message);
Evaluating Comparisons Among Different Data Types
JavaScript allows comparison between different data types, which can lead to type coercion. It’s crucial to understand how these comparisons work to avoid unexpected results.
Special Cases and Considerations When comparing a string with a number, JavaScript converts the string to a number. For example:
let result = "5" > 3;
console.log(result); // true, as "5" becomes 5
In cases involving null
and undefined
, special rules apply. For equality:
console.log(null == undefined); // true
However, for non-equality:
console.log(null != undefined); // false
Advanced JavaScript Operators and Their Applications
Introduction to the Nullish Coalescing Operator (??)
The Nullish Coalescing Operator, denoted by ??
, is a logical operator introduced in ES2020. It returns the right-hand operand when the left-hand operand is null
or undefined
, otherwise the left-hand operand. This operator is particularly useful in handling default values.
Explanation and Purpose
In JavaScript, logical operators like ||
have often been used to provide default values. However, ||
returns the right-hand operand for any falsy value of the left-hand operand, including 0
, ''
(empty string), and false
. The ??
operator overcomes this limitation by only considering null
or undefined
as values that trigger the use of the right-hand operand.
Illustrative Example of Using ??
Suppose you have a function that sets up user profiles, and you want to assign a default age when it is not provided:
function setupUserProfile(name, age) {
let userAge = age ?? 30;
console.log(`Name: ${name}, Age: ${userAge}`);
}
setupUserProfile("Alice", null); // Outputs: "Name: Alice, Age: 30"
setupUserProfile("Bob", 25); // Outputs: "Name: Bob, Age: 25"
In this example, when age
is null
, the default age of 30 is used.
Understanding the Optional Chaining Operator (?.)
The Optional Chaining Operator, represented by ?.
, allows you to read the value of a property located deep within a chain of connected objects without having to check each reference in the chain.
Conceptual Overview
Optional chaining is a safe way to access nested object properties, even if an intermediate property doesn’t exist. It prevents errors that might otherwise occur when attempting to access properties of undefined
or null
values.
Practical Application Example
Consider an object representing a user, which may or may not have information about the user’s address:
let user = {
name: "John",
address: { street: "123 Main St", city: "Anytown" }
};
console.log(user.address?.street); // "123 Main St"
console.log(user.contact?.email); // undefined
In this case, attempting to access user.contact.email
would typically result in an error if contact
is not defined. However, with the optional chaining operator ?.
, it safely returns undefined
.
Conclusion
In conclusion, JavaScript’s not equal and other comparison operators play a pivotal role in effective coding, enabling developers to make precise and logical decisions within their scripts. Understanding these operators, including the nuances of the nullish coalescing and optional chaining operators, is crucial for writing robust, error-free code. By mastering these elements, developers can harness the full potential of JavaScript, ensuring their applications are both functional and efficient. The journey through JavaScript’s comparison landscape reveals the language’s flexibility and depth, making it an indispensable skill for any aspiring or seasoned programmer.
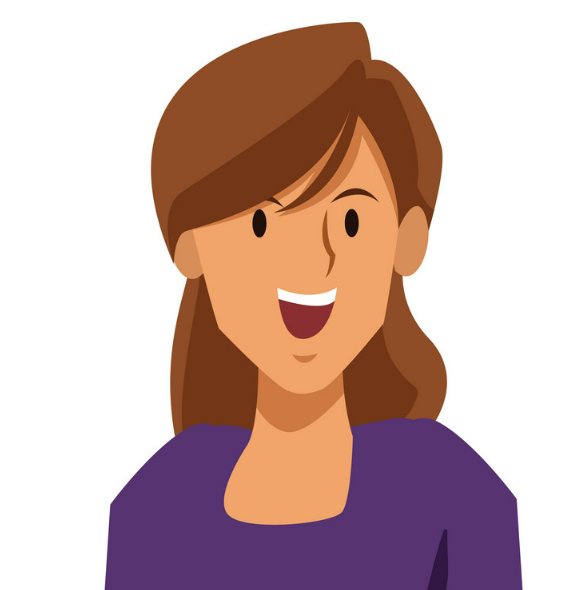
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.