Introduction: Understanding HTML to String Conversion in JavaScript
The process of converting HTML to a string in JavaScript is more than just a technical procedure; it’s an essential part of modern web development. This capability is crucial for developers who need to dynamically manipulate web content. For instance, when a web application needs to update its content based on user interaction or an external event, converting HTML elements to strings becomes necessary. This conversion enables the JavaScript code to handle HTML elements as string data, which can then be easily manipulated, stored, or sent over a network.
Moreover, this conversion plays a significant role in enhancing the functionality and responsiveness of web applications. A responsive web application responds to user inputs in real-time, and converting HTML to strings is often a part of this interactive process. For example, when a user submits a form, the form’s HTML elements can be converted to strings for validation, processing, or sending the data to a server. This dynamic interaction is a key feature of modern web applications, making HTML to string conversion an indispensable tool in a developer’s arsenal.
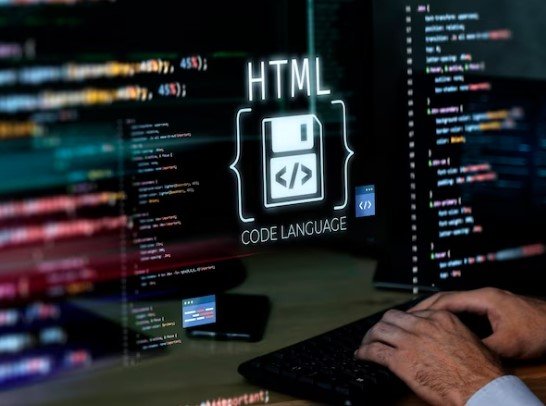
Overview of HTML to Plain Text Conversion
The conversion of HTML to plain text is a specific type of transformation that involves stripping HTML tags and leaving only the readable text. This process is vital in several scenarios, especially in data extraction and content manipulation. For example, when scraping data from a web page or processing content for display in a non-HTML environment, converting HTML to plain text becomes necessary.
This conversion is not just about removing HTML tags; it’s about preserving the essential text in a format that is easily readable and usable. In scenarios where the presence of HTML tags could disrupt the functionality or the intended display of content, such as in text-only email clients or simple text viewers, converting HTML to plain text ensures that the content remains accessible and meaningful.
Comprehensive Methods to Convert HTML into String
Method 1: Using Regular Expressions with .replace()
Approach:
- This method involves using the
.replace()
method in conjunction with regular expressions. - It strips HTML tags from a string, leaving only the text content.
Usage:
- Commonly used for simple HTML structures.
- Effective for quick removal of tags without the need for DOM manipulation.
Example:
let htmlString = "<p>Hello, <b>world</b>!</p>"; let textString = htmlString.replace(/<[^>]*>/g, "");
Method 2: Creating and Using Temporary DOM Elements
Approach:
- Create a temporary DOM element in JavaScript.
- Insert the HTML content into this element.
- Use
textContent
orinnerText
properties to extract plain text.
Usage:
- Handles complex HTML structures accurately.
- Ensures correct parsing of HTML.
Example:
let tempDiv = document.createElement("div");
tempDiv.innerHTML = htmlString;
let plainText = tempDiv.textContent || tempDiv.innerText;
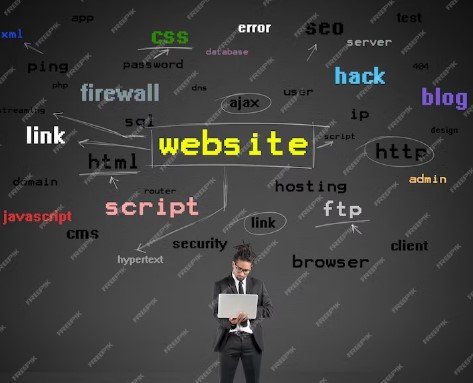
Method 3: Implementing the html-to-text
npm Package
Approach:
- Utilize the
html-to-text
package in Node.js applications. - Converts HTML into a string with several customization options.
Usage:
- Ideal for server-side applications in Node.js.
- Handles various HTML structures and elements efficiently.
Example:
const htmlToText = require('html-to-text');
let text = htmlToText.fromString(htmlString, { wordwrap: 130 });
Retrieving the Full HTML Document as a String
Approach:
- Use JavaScript methods like
document.documentElement.outerHTML
.
Usage:
- Retrieves the entire HTML source of a webpage as a string.
- Useful for scenarios requiring the complete HTML structure.
Example:
let fullHTMLString = document.documentElement.outerHTML;
JavaScript getElementsByTagName()
Method
Approach:
- Select elements by their tag name using
getElementsByTagName()
.
Usage:
- Simplifies access and manipulation of specific parts of the HTML document.
Example:
let paragraphs = document.getElementsByTagName("p");
Employing the HTML DOM innerHTML
Property
Approach:
- Use the
innerHTML
property to retrieve or set the HTML markup inside an element.
Usage:
- Directly accesses and modifies the HTML structure.
- Retrieves HTML as a string from a specific element.
Example:
let divContent = document.getElementById("myDiv").innerHTML;
Conclusion
Converting HTML to a string in JavaScript is essential for web development, enhancing the ability to manipulate web content. By understanding and using different methods, such as regular expressions, DOM manipulation, and specific npm packages, developers can efficiently handle HTML-to-string conversion in a variety of scenarios. Each method has its use cases and advantages, making it important to choose the right approach based on the specific needs of the project.
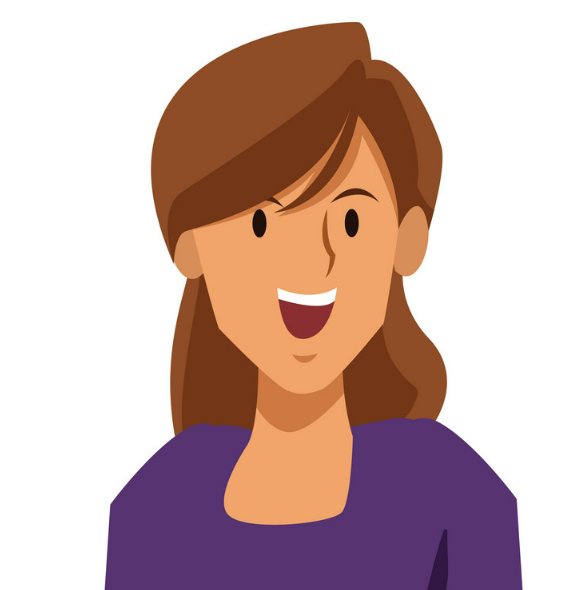
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.