Introduction
ReactJS stands as a pivotal cornerstone in the domain of web development, renowned for its efficiency and flexibility. This JavaScript library, developed by Facebook, has revolutionized the way developers construct user interfaces, especially for single-page applications. Its component-based architecture allows for the creation of reusable UI components, thus facilitating the development process and enhancing the overall performance of web applications.
Understanding REST APIs: A Primer
REST, or Representational State Transfer, APIs have become the backbone of web communications in modern development paradigms. These APIs are designed around the principles of REST, a software architectural style that defines a set of constraints for creating web services. REST APIs operate on the premise of making networked resources available through predictable URLs, emphasizing a stateless communication protocol between the client and the server. This design approach ensures that RESTful services are scalable, simple, and lightweight, making them an ideal choice for web application development.
The essence of REST APIs lies in their use of standard HTTP methods to perform operations on resources. These methods—GET, POST, PUT, DELETE, and PATCH—play a crucial role in defining the interaction between the client and the server. Each method corresponds to a specific operation: retrieving data, creating a new resource, updating an existing resource, deleting a resource, and partially updating a resource, respectively. This standardized use of HTTP methods facilitates a uniform interface for accessing and manipulating web resources, thereby simplifying the development process and enhancing the interoperability between different systems and applications.
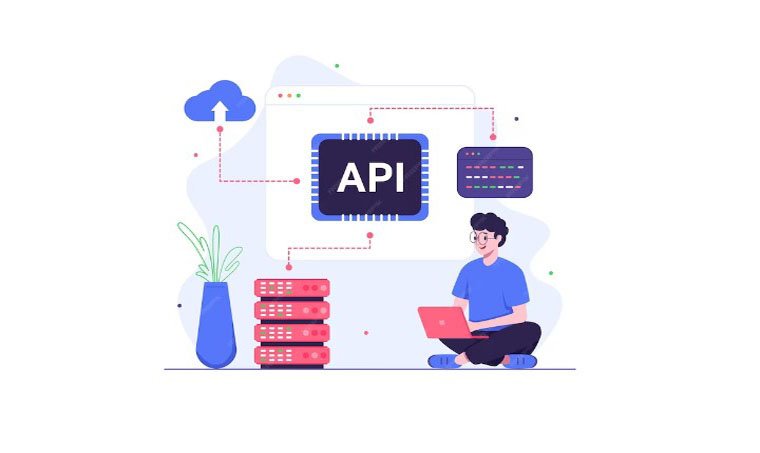
Setting Up Your Project Foundation
Configuring Your React Environment
To kickstart your React project, setting up a solid foundation is paramount. This begins with configuring your React environment, which can be effortlessly accomplished using Create React App (CRA), a widely recognized scaffolding tool provided by the React team. CRA abstracts the complexity of tool configuration (Webpack, Babel, etc.) and allows you to dive straight into development. To initiate a new React project, execute the following command in your terminal:
npx create-react-app my-react-app
This command creates a new directory named my-react-app
with all the necessary setup for a React application. Once the setup is complete, you can start the development server with
cd my-react-app
npm start
The npm start
command runs the app in development mode, opening it in your default web browser.
Essential Tools and Libraries for API Integration
Integrating APIs into your React application requires a set of tools and libraries that streamline the process of making HTTP requests and handling responses. One of the most fundamental tools is fetch
, a native web API for making asynchronous requests. However, for enhanced features and simplicity, libraries like Axios can be advantageous.
Axios is a promise-based HTTP client for the browser and node.js, offering an easy-to-use API and automatic transformations of JSON data. To include Axios in your project, install it via npm:
npm install axios
Axios simplifies making HTTP requests, handling responses, and catching errors, making it an invaluable tool for API integration in your React projects.
Accessing and Managing Data
Accessing REST APIs within React Applications
To access REST APIs within your React application, you first need to set up the environment for making API calls. This involves choosing the right library or tool and understanding how to incorporate it into your React components.
Setting Up the Environment for Making API Calls
Using the Axios library as an example, the environment setup for making API calls is straightforward. After installing Axios, you can start making requests to your chosen API by importing Axios into your React component:
import axios from 'axios';
Then, you can use Axios to make GET requests, POST requests, or any other HTTP operations your application requires. Here’s a simple example of making a GET request to fetch data from an API:
componentDidMount() {
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
// Handle the data received from the API
})
.catch(error => {
console.error("There was an error fetching the data: ", error);
// Handle any errors encountered during the request
});
}
This snippet demonstrates how to fetch data in a React component lifecycle method, componentDidMount
, ensuring that the API call is made when the component is mounted to the DOM.
Utilizing the Fetch API for API Integration
Introduction to the Fetch API
The Fetch API is a modern interface that allows web developers to make HTTP requests to servers from web browsers. Unlike older techniques such as XMLHttpRequest, the Fetch API provides a more powerful and flexible feature set to work with. It returns promises, making it easier to work with asynchronous operations and to manage multiple concurrent requests. The Fetch API supports the entire HTTP protocol, so developers can perform GET, POST, PUT, DELETE, and other requests in a straightforward manner.
Basic Setup and Syntax
The basic syntax of the Fetch API is simple and easy to understand. At its most basic, a fetch request requires only one argument — the URL of the resource you wish to fetch. Here is a simple example:
fetch('https://api.example.com/data')
.then(response => response.json()) // Converts the response to JSON
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
This code snippet fetches data from the specified URL and then processes the response as JSON. If the request is successful, it logs the data to the console. If there is an error in the request, it catches the error and logs an error message.
Executing GET Requests in React with the Fetch API
Step-by-step Guide to Performing GET Requests
To perform a GET request in a React component, you can use the componentDidMount
lifecycle method or the useEffect
hook in functional components. This ensures that the fetch request is made when the component is rendered. Here’s an example using useEffect
in a functional component:
import React, { useState, useEffect } from 'react';
function FetchDataComponent() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => setData(data))
.catch(error => console.error('Error fetching data:', error));
}, []); // The empty array means this effect runs once after the initial render
return (
<div>
{data ? <pre>{JSON.stringify(data, null, 2)}</pre> : 'Loading data...'}
</div>
);
}
This component fetches data from an API and stores it in the state. If the data is successfully fetched, it is displayed; otherwise, a loading message is shown.
Best Practices and Error Handling
When using the Fetch API, especially in a React application, it’s important to handle errors gracefully. This includes checking for HTTP error statuses and catching any errors that occur during the fetch operation. Implementing error handling ensures that your application can respond to issues like network errors or server problems without crashing.
Implementing POST Requests in React via Fetch API
How to Send Data to a Server Using POST Requests
To send data to a server using a POST request, you can specify an options object as the second argument to fetch
, providing the method
, headers
, and body
properties:
function postData(url = '', data = {}) {
return fetch(url, {
method: 'POST', // *GET, POST, PUT, DELETE, etc.
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
})
.then(response => response.json());
}
// Example POST method implementation:
postData('https://api.example.com/data', { answer: 42 })
.then(data => console.log(data)) // JSON data parsed by `response.json()` call
.catch(error => console.error('Error:', error));
This function sends a POST request to the specified URL with the data provided as JSON. It then parses the JSON response from the server.
Handling Server Responses
After sending a POST request, it’s crucial to handle the server’s response appropriately. The server might return data that needs to be processed, or there could be error statuses that need to be addressed. Always check the response status and use the data returned by the server as needed in your application. Properly handling server responses ensures that your application can react correctly to the outcome of the POST request, whether it’s successful or there’s an error.
Deleting Data with the Fetch API in React
Guide to Implementing DELETE Requests
Implementing DELETE requests with the Fetch API in React involves sending a request to a server endpoint designated for deleting a specific resource. This is commonly done in response to user actions, such as clicking a delete button next to an item in a list. Here’s how you can structure a DELETE request using Fetch:
const deleteData = async (url = '') => {
try {
const response = await fetch(url, {
method: 'DELETE', // Specify the DELETE method
});
// Check if the deletion was successful
if (!response.ok) {
throw new Error('Error deleting the resource');
}
console.log('Resource deleted successfully');
} catch (error) {
console.error('Error:', error);
}
};
// Example usage
deleteData('https://api.example.com/items/123');
Managing State After Deletion
After successfully deleting data on the server, it’s important to update the application’s state to reflect this change. This ensures the UI is in sync with the backend data. If you’re using React hooks, you might manage state with useState
and update it after a successful deletion:
const [items, setItems] = useState([...]);
const handleDelete = async (itemId) => {
await deleteData(`https://api.example.com/items/${itemId}`);
// Update the items state by filtering out the deleted item
setItems(currentItems => currentItems.filter(item => item.id !== itemId));
};
This approach filters out the item from the local state, removing it from the UI without needing to fetch the entire list again from the server.
Mastering Async/Await for Fetch API Calls
Simplifying Asynchronous API Calls with Async/Await
Async/Await syntax in JavaScript simplifies working with promises, making asynchronous code look more like synchronous code. This is particularly useful with the Fetch API for making API calls. Here’s a basic example of using Async/Await with Fetch to make a GET request:
async function fetchData(url = '') {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
return data;
} catch (error) {
console.error('There was an error:', error);
}
}
// Example usage
fetchData('https://api.example.com/data').then(data => console.log(data));
This function asynchronously fetches data from the provided URL and returns the parsed JSON. The use of Async/Await makes the flow of asynchronous requests and error handling clearer and more intuitive.
Error Handling in Asynchronous Operations
Error handling is a critical part of working with asynchronous operations, especially when using Async/Await with the Fetch API. The try/catch block is effectively used to catch any errors that occur during the fetch operation or while processing the response. This approach ensures that your application can gracefully handle network errors, bad responses, and data processing issues:
async function safeFetchData(url = '') {
try {
const response = await fetch(url);
// Ensure the fetch was successful
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Fetch error:', error);
// Handle the error according to your application's needs
}
}
This pattern allows you to manage both the asynchronous nature of network requests and the need for robust error handling in a clear and structured way, making your React application more reliable and user-friendly.
Enhancing Functionality and User Experience
Interactive Guide: Step-by-Step API Integration
Building a simple project to demonstrate API integration not only helps in understanding the process but also showcases the power of React combined with Fetch API. Let’s create a basic React application that fetches and displays data from an API.
Building a Simple Project to Demonstrate API Integration
Consider a project that fetches user data from a public API and displays it. We’ll use https://jsonplaceholder.typicode.com/users
as our API endpoint for demonstration.
First, set up your React project environment if you haven’t already:
npx create-react-app api-integration-demo
Next, create a component that fetches and displays users:
import React, { useState, useEffect } from 'react';
function UsersList() {
const [users, setUsers] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
async function fetchUsers() {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
const data = await response.json();
setUsers(data);
setLoading(false);
}
fetchUsers().catch(error => console.error('Error fetching users:', error));
}, []);
if (loading) return <div>Loading...</div>;
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
This component uses the useEffect
hook to fetch user data when the component mounts and updates the component’s state with the fetched data, displaying a simple list of user names.
Incorporating React Components and Managing State
React’s component-based architecture and state management capabilities make it ideal for building dynamic interfaces that respond to data changes. In the UsersList
component, useState
is used to manage the users’ list and loading state, ensuring that the UI updates reactively as data is fetched and loaded.
Managing Fetch API Errors Gracefully
Handling errors in API calls is crucial for a good user experience. Let’s improve our UsersList
component to handle errors more gracefully.
Strategies for Robust Error Handling
A robust error handling strategy involves catching errors that occur during the API call and providing feedback to the user. Modify the fetchUsers
function to include try-catch blocks:
useEffect(() => {
async function fetchUsers() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
setUsers(data);
} catch (error) {
console.error('Error fetching users:', error);
} finally {
setLoading(false);
}
}
fetchUsers();
}, []);
Displaying Error Messages to Users
To improve the user experience, display an error message when an error occurs:
const [error, setError] = useState('');
// Inside fetchUsers, set the error state in the catch block
catch (error) {
setError('Failed to fetch users');
console.error('Error fetching users:', error);
}
// In the component's return statement, conditionally render the error message
return (
<div>
{error && <p>{error}</p>}
{loading ? <div>Loading...</div> : (
<ul>
{users.map(user => <li key={user.id}>{user.name}</li>)}
</ul>
)}
</div>
);
Understanding HTTP Status Codes
Interpreting HTTP status codes correctly is essential for handling responses from the Fetch API effectively.
Interpreting Common HTTP Status Codes in the Context of API Calls
- 200 OK: The request has succeeded, and the response contains the requested data.
- 201 Created: The request has succeeded, and a new resource has been created as a result.
- 400 Bad Request: The server cannot process the request due to a client error (e.g., malformed request syntax).
- 401 Unauthorized: Authentication is required, and it has either failed or has not been provided.
- 404 Not Found: The server cannot find the requested resource.
- 500 Internal Server Error: The server encountered an unexpected condition that prevented it from fulfilling the request.
Incorporating checks for these status codes in your Fetch API calls helps in providing appropriate feedback to the user and handling errors and successes appropriately:
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
By understanding and handling these status codes, you can create more robust and user-friendly web applications that communicate effectively with APIs.
Rendering API Data in React Views
Techniques for Displaying API Data Effectively
Displaying API data effectively in React involves understanding how to integrate fetched data into your React components’ state and render it in the UI. A common approach is to fetch data in a lifecycle method or hook and then use the component’s state to store this data. Here’s a basic pattern using class components:
import React, { Component } from 'react';
class UsersList extends Component {
constructor(props) {
super(props);
this.state = {
users: [],
isLoading: true,
};
}
componentDidMount() {
fetch('https://api.example.com/users')
.then(response => response.json())
.then(data => this.setState({ users: data, isLoading: false }))
.catch(error => console.error('Fetching users failed:', error));
}
render() {
const { users, isLoading } = this.state;
return (
<div>
{isLoading ? <p>Loading...</p> : (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
)}
</div>
);
}
}
This component fetches user data when it mounts and stores this data in its state, which is then used to render a list of users.
Utilizing componentDidMount for Initial Data Fetching
The componentDidMount
lifecycle method is ideal for fetching data in class components. This method runs after the component output has been rendered to the DOM, making it the perfect place to fetch data:
componentDidMount() {
fetch('https://api.example.com/users')
.then(response => response.json())
.then(data => this.setState({ users: data, isLoading: false }))
.catch(error => console.error('Fetching users failed:', error));
}
Enhancing User Experience
Tips for Improving UI/UX in API-driven Applications
Improving UI/UX in API-driven applications involves several strategies to make the application more responsive and user-friendly. Some key strategies include:
- Optimistic Updates: Update the UI optimistically after user actions, then adjust based on the server’s response. This makes the app feel faster and more responsive.
- Caching Responses: Cache API responses to reduce loading times and decrease the number of API calls, improving the overall performance.
- Error Feedback: Provide clear and helpful error messages to users when things go wrong, helping them understand what happened and how to proceed.
Loading Indicators and Data Refresh Strategies
Implementing loading indicators and effective data refresh strategies can significantly enhance the user experience by keeping the user informed about the application’s state. Here’s how you can implement a loading indicator:
const { users, isLoading } = this.state;
return (
<div>
{isLoading ? <p>Loading...</p> : (
<ul>
{users.map(user => <li key={user.id}>{user.name}</li>)}
</ul>
)}
</div>
);
For data refresh, you could implement a button that, when clicked, triggers a data refetch:
<button onClick={this.fetchData}>Refresh Data</button>
Practical Applications and Advanced Topics
Real-world Scenarios and How to Handle Them
In real-world scenarios, you might need to make API calls to perform CRUD operations. Here’s an example of how to handle creating a new user via a POST request:
async function createUser(userData) {
try {
const response = await fetch('https://api.example.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(userData),
});
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
console.log('User created successfully');
return await response.json();
} catch (error) {
console.error('Error creating user:', error);
}
}
Interactive Examples with Code Snippets
For an interactive example, consider a form in your React application that allows users to submit data, which is then sent to a server:
class UserForm extends React.Component {
state = { userName: '' };
handleSubmit = async (event) => {
event.preventDefault();
await createUser({ name: this.state.userName });
this.setState({ userName: '' }); // Reset the form field
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<input
type="text"
value={this.state.userName}
onChange={e => this.setState({ userName: e.target.value })}
placeholder="Enter a username"
required
/>
<button type="submit">Create User</button>
</form>
);
}
}
This form captures user input, allowing them to enter a username that is then submitted to a server via a POST request when the form is submitted. This kind of interactive example demonstrates how to integrate API calls into a React application for a dynamic user experience.
Additional Considerations for API Integration
Security Best Practices
When integrating APIs into your React applications, security should be a top priority. A common best practice is to use environment variables to store sensitive information such as API keys or URLs. This approach ensures that your sensitive data is not hard-coded into your application’s source code, reducing the risk of exposing it in your version control system or to the public. Here’s how you can set up and use environment variables in a React app:
- Create a
.env
file in the root of your project. - Add environment variables prefixed with
REACT_APP_
, like so:
REACT_APP_API_URL=https://api.example.com
REACT_APP_API_KEY=yourapikey
Access these variables in your application using process.env
:
const apiUrl = process.env.REACT_APP_API_URL;
const apiKey = process.env.REACT_APP_API_KEY;
This method provides a secure way to use and manage sensitive information in your React applications.
Dealing with CORS and Other Common Challenges
Cross-Origin Resource Sharing (CORS) is a security feature that restricts how resources on a web server can be requested from another domain. When making API calls from a React application hosted on a different domain than the API, you might encounter CORS errors. To handle CORS:
- Server-Side: Ensure the server is configured to accept requests from your application’s domain. This typically involves setting the
Access-Control-Allow-Origin
header to your domain or*
(which should be used with caution). - Development: Use a proxy in your development environment, such as setting up a proxy in the
package.json
of your React app:
"proxy": "https://api.example.com",
This setup tells the development server to proxy requests to the specified API, helping to bypass CORS issues during development.
Conclusion: Wrapping Up
Key Takeaways from the Tutorial
Throughout this tutorial, we’ve explored the essentials of integrating APIs in React applications, covering everything from setting up your React environment, making GET and POST requests, to handling errors and displaying data. We’ve also discussed security best practices and how to manage CORS issues, which are critical for building secure and functional web applications.
Best Practices and Final Thoughts on API Integration in ReactJS
API integration in ReactJS requires careful consideration of various aspects, including security, error handling, and user experience. Here are some final thoughts and best practices to keep in mind:
- Security: Always use environment variables for sensitive information and ensure your API endpoints are secure.
- Error Handling: Implement robust error handling to improve the user experience and make your application more resilient.
- User Experience: Use loading indicators and manage state effectively to ensure your application is responsive and user-friendly.
- CORS: Understand and appropriately handle CORS to ensure smooth communication between your React application and the server.
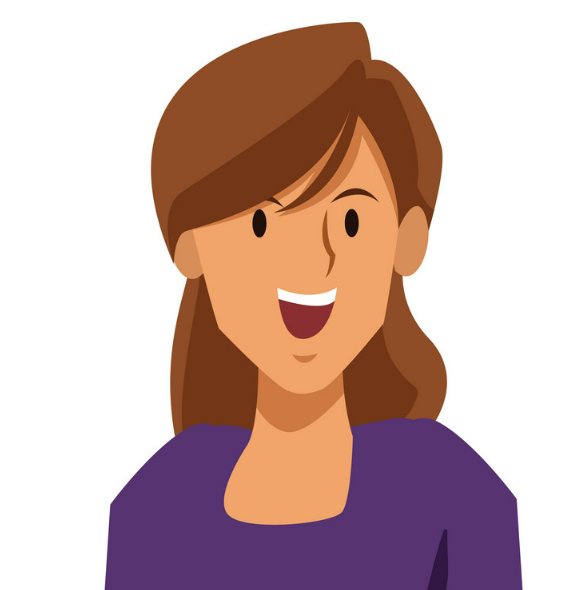
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.