Introduction
Angular, a platform and framework for building single-page client applications using HTML and TypeScript, has been a staple in web development for creating dynamic and interactive web applications. The HttpClient
module, introduced in Angular, revolutionizes how developers interact with external APIs by providing a more powerful and flexible way to make HTTP requests. Angular 10, the latest version at the time, brings new features and improvements that enhance performance, development efficiency, and the overall experience of building Angular applications.
Angular 10: New Features and Improvements
Angular 10 introduces several new features and improvements, including stricter settings, new date range picker in the Angular Material UI component library, and several new compiler options for more advanced debugging and better performance. These updates aim to streamline the development process and offer developers more control and precision.
Getting Started with Angular 10
Setting up the Angular environment and creating your first project in Angular 10 involves a few essential steps, ensuring you have the necessary tools and understanding to start developing robust applications.
Setting Up the Angular Environment: Prerequisites and Installation Steps
Before diving into Angular 10, ensure that Node.js is installed on your system, as it comes with npm (node package manager) used for managing Angular’s dependencies. With Node.js installed, you can install the Angular CLI globally using npm:
npm install -g @angular/cli
This command installs the Angular CLI, which is instrumental in creating projects, generating application and library code, performing development tasks such as testing, bundling, and deployment.
Creating Your First Angular Project: A Simple Walkthrough
To create your first Angular project, execute the following command in your terminal:
ng new my-first-angular-app
This command prompts you for some configurations like whether to add Angular routing and which stylesheet format you prefer. Once the project is created, navigate into your project directory and serve your application:
cd my-first-angular-app
ng serve
This command compiles the application and starts a web server. By navigating to http://localhost:4200/
, you can see your new Angular application running.
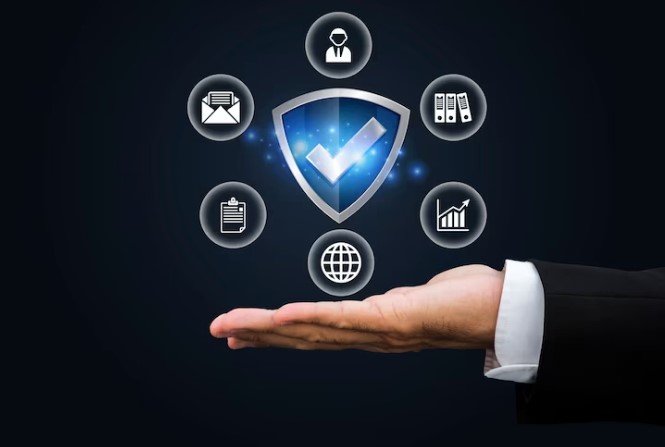
Integrating HttpClient into Angular’s Core
HttpClient
provides a powerful way to communicate with backend services over HTTP.
Understanding HttpClient: Why It’s a Game-Changer for API Interactions
HttpClient
offers a significant advantage for API interactions by supporting typed request and response objects, interceptors for request and response transformation, and streamlined error handling. These features make it an indispensable tool for modern web development in Angular.
Step-by-Step Guide to Importing HttpClient into Your Angular Application
To use HttpClient
, you first need to import HttpClientModule
in your app’s main module (app.module.ts):
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
// other components
],
imports: [
BrowserModule,
HttpClientModule // import HttpClientModule here
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Incorporating HttpClient in Components or Services
HttpClient
is most effectively used within Angular services or components to interact with APIs.
How to Inject HttpClient in Angular Services for API Interactions
To use HttpClient
in a service, first, import and inject it into your service class:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class DataService {
constructor(private http: HttpClient) { }
fetchData() {
return this.http.get('https://api.example.com/data');
}
}
Best Practices for Organizing Your Modules and Components
Organizing your Angular modules and components effectively is crucial for maintainability and scalability. Keep related components and services together, lazy load modules to improve startup time, and group your service calls within dedicated services rather than directly in components.
Executing API Requests: The Essentials
Performing API requests is a fundamental aspect of web development, allowing your application to interact with external data sources.
The Basics of HTTP in Angular: GET, POST, PUT, DELETE Methods
Angular’s HttpClient
provides methods for all HTTP requests:
// GET request
this.http.get('/api/items');
// POST request
this.http.post('/api/items', { name: 'NewItem' });
// PUT request
this.http.put('/api/items/1', { name: 'UpdatedItem' });
// DELETE request
this.http.delete('/api/items/1');
Constructing Query Parameters and Headers Effectively
To send query parameters and headers with your requests, use the options object:
this.http.get('/api/items', {
params: { filter: 'recent' },
headers: { Authorization: 'Bearer your-token-here' }
});
Designing a Robust Error Handling Mechanism for Your API Calls
Error handling in Angular can be implemented using RxJS operators such as catchError
within your service methods:
import { catchError } from 'rxjs/operators';
import { throwError } from 'rxjs';
fetchData() {
return this.http.get('https://api.example.com/data').pipe(
catchError(error => {
console.error('Error fetching data', error);
return throwError(error);
})
);
}
Implementing these practices in your Angular applications ensures efficient API interactions, organized codebase, and a robust error handling mechanism, setting a strong foundation for developing dynamic and interactive web applications.
Advanced HttpClient Usage
Angular’s HttpClient
offers a suite of advanced features designed to enhance the functionality and efficiency of HTTP communications in web applications. By leveraging these features, developers can create more dynamic, responsive, and user-friendly applications.
Leveraging Advanced Features of HttpClient for Enhanced Functionality
One of the advanced features of HttpClient
includes the use of HTTP interceptors, which allow developers to manipulate HTTP requests and responses globally. This can be useful for adding headers to every request, logging requests, or handling errors consistently across the application.
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable()
export class MyInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const modifiedReq = req.clone({
headers: req.headers.set('Authorization', `Bearer your-token-here`),
});
return next.handle(modifiedReq);
}
}
Strategies for Error and Exception Management in API Interactions
Effective error and exception management ensures that applications can handle unexpected situations gracefully, maintaining a smooth user experience. Implementing a global error handler with interceptors can help manage API errors centrally.
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
return next.handle(req).pipe(
catchError((error: HttpErrorResponse) => {
console.error(`Error occurred: ${error.message}`);
return throwError(error);
})
);
}
Optimizing Performance with Caching Techniques and HTTP Interceptors
Caching responses from API calls can significantly improve the performance of Angular applications by reducing the number of necessary HTTP requests. HTTP interceptors can be used to implement caching by storing responses and returning cached data for identical requests.
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const cachedResponse = this.cache.get(req.url);
return cachedResponse ? of(cachedResponse) : next.handle(req).pipe(
tap(event => {
if (event instanceof HttpResponse) {
this.cache.set(req.url, event);
}
})
);
}
File Uploads and Data Management
Handling file uploads and managing data efficiently are crucial aspects of modern web applications, enhancing the user experience and functionality.
Handling File Uploads Through API Calls Using FormData
For file uploads, Angular applications can utilize FormData
to send files to a server. This is particularly useful for POST requests where files need to be uploaded alongside other data.
uploadFile(file: File): Observable<any> {
const formData: FormData = new FormData();
formData.append('file', file, file.name);
return this.http.post('/api/upload', formData, {
reportProgress: true,
observe: 'events'
});
}
Mastering the Dynamics of POST Requests and Sending Data Efficiently
Efficiently managing POST requests is key to optimizing data transmission. Angular’s HttpClient
allows for the sending of structured data as JSON, making it straightforward to interact with RESTful APIs.
createItem(item: Item): Observable<Item> {
return this.http.post<Item>('/api/items', item);
}
Testing and Reliability
Ensuring the reliability of API calls is paramount in developing robust Angular applications. Angular provides tools specifically designed for testing HTTP interactions.
Ensuring API Call Reliability: An Introduction to HttpClientTestingModule
HttpClientTestingModule
simplifies the testing of HTTP requests by mocking the HttpClient
. This allows for the simulation of different scenarios without the need for an actual backend.
import { TestBed } from '@angular/core/testing';
import { HttpClientTestingModule, HttpTestingController } from '@angular/common/http/testing';
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [DataService]
});
});
Best Practices for Testing API Calls in Angular Applications
When testing API calls, it’s important to simulate responses and verify that your application behaves as expected. Using HttpTestingController
, you can ensure that your service correctly handles both successful responses and errors.
it('should fetch data successfully', () => {
const testData: Data = { /* Mock data */ };
const httpTestingController = TestBed.get(HttpTestingController);
dataService.fetchData().subscribe(data => {
expect(data).toEqual(testData);
});
const req = httpTestingController.expectOne('/api/data');
req.flush(testData);
});
Comparative Analysis and Insights
Understanding the different tools and techniques available for making HTTP requests in Angular is crucial for selecting the right approach for your application.
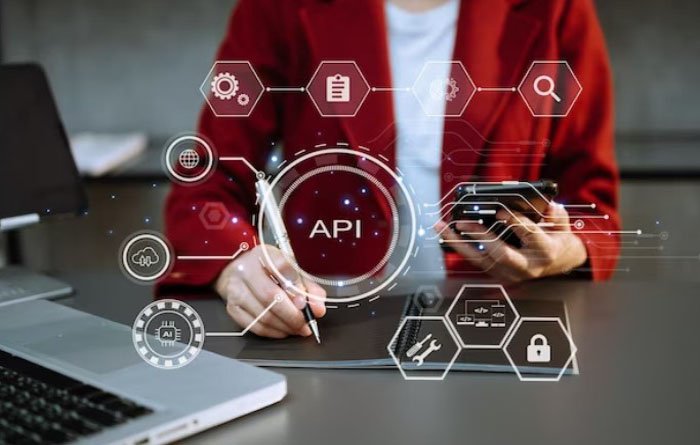
HttpClient vs. HttpBackend: Understanding the Differences and Use Cases
HttpClient
is a higher-level service that provides a simple API for HTTP requests, automatically handling JSON data serialization and response type casting. HttpBackend
is a lower-level service that HttpClient
uses to make requests, offering more control but requiring more boilerplate code for handling responses.
Insights into the Significance of API Calls in Angular Applications
API calls are the backbone of data-driven Angular applications, enabling dynamic content, user authentication, and interaction with external services. Mastering HttpClient
and understanding its underlying mechanisms are essential for developing sophisticated web applications.
Broadening Your Angular Knowledge
Expanding your Angular skills can open up new career opportunities and enhance your ability to create advanced web applications.
Enhancing Your Coding Skills with UpGrad’s Software Engineering Courses
UpGrad offers a variety of software engineering courses, including specialized programs in full-stack development, that can help deepen your understanding of Angular and other modern web technologies.
Opportunities to Pursue a Career as a Full-Stack Developer with Certifications from Prestigious Institutions
Certifications from prestigious institutions can validate your skills and make you more attractive to employers. Pursuing specialized training and certification in Angular and full-stack development can be a significant career boost.
Conclusion: Wrapping Up and Key Takeaways
Mastering HttpClient
in Angular is crucial for developers looking to build dynamic, data-driven web applications. This guide has covered the essentials of making HTTP requests, managing data, and ensuring the reliability and performance of your Angular applications. By understanding the advanced features of HttpClient
, implementing efficient data management techniques, and adhering to best practices for testing, developers can create robust, efficient, and user-friendly web applications. As you continue to explore Angular and its ecosystem, remember the importance of continuous learning and staying updated with the latest trends and technologies.
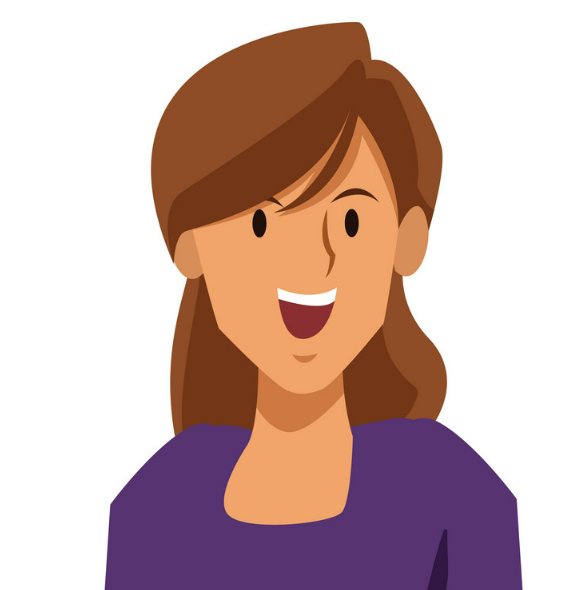
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.