Overview of App Categories
Introduction to Shopify Apps
Shopify apps extend the functionality of Shopify stores, enabling merchants to add custom features, integrate with other software, and improve their e-commerce operations. There are three main types of Shopify apps: public, private, and custom. Public apps are available in the Shopify App Store and can be used by any Shopify merchant. They must meet Shopify’s approval standards and are designed for a broad user base. Private apps are developed for a specific Shopify store or merchant. These apps are not listed in the Shopify App Store and offer a tailored solution to meet the unique needs of a single merchant or a small number of merchants. Custom apps, introduced more recently, combine elements of both public and private apps. They are built for specific use cases but can be distributed to multiple merchants through unique installation links, providing a middle ground between the wide availability of public apps and the tailored specificity of private apps.
Choosing the Right App for Your Needs
Selecting the right app category for your Shopify store depends on your specific needs, budget, and the level of customization required. Public apps are ideal for common e-commerce functionalities like SEO maintenance, or inventory management that many stores require. These apps are often less expensive and quicker to implement than developing a custom solution. Private apps suit merchants with unique business processes that cannot be addressed with off-the-shelf apps. They require more investment but offer tailored solutions that closely fit the merchant’s operations. Custom apps are suitable for businesses that need a specialized app that can be shared with a specific group of stores, offering a balance between customization and distribution.
Preparatory Steps
Setting Up Your Development Environment
Before beginning the development of a Shopify app, it’s essential to set up a proper development environment. This setup includes installing necessary software development tools, such as code editors (e.g., Visual Studio Code), version control systems (e.g., Git), and the Shopify CLI (Command Line Interface), which is crucial for creating, testing, and deploying Shopify apps. Additionally, developers should have a Shopify Partner account, which provides access to development stores for testing apps in a live environment. Understanding the fundamentals of web development, including HTML, CSS, JavaScript, and backend programming languages like Ruby or Python, is also crucial.
Understanding Shopify API Requirements
Developing Shopify apps requires a deep understanding of the Shopify API, which serves as the backbone for interacting with Shopify stores programmatically. The Shopify API offers a wide range of endpoints for various functionalities, including product management, order processing, and customer data manipulation. Developers must familiarize themselves with the API’s rate limits, authentication mechanisms (such as OAuth for public apps), and data security practices. It’s also important to stay updated with the latest API versions and changes, as Shopify frequently updates its platform to introduce new features and improvements.
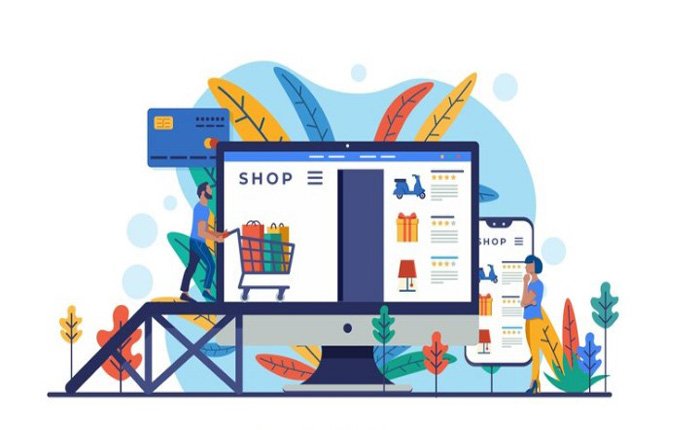
Procedure for Generating an Access Token
Initial Phase: Collecting Store Information
The first step in generating an access token for a Shopify app involves collecting essential information from the store you’re planning to integrate with. This information typically includes the store’s unique Shopify domain (e.g., storename.myshopify.com
) and the permissions or scopes your app needs to function correctly. These scopes define the level of access to the store’s data your app is requesting, such as reading orders, modifying products, or accessing customer information. Understanding precisely what information your app needs to operate and what permissions are required is crucial for a smooth integration process.
Phase 2: Securing Installation Consent
Crafting the Installation URL
After gathering the necessary store details, the next step is to obtain installation consent from the store owner. This is done by crafting a URL that directs the store owner to Shopify’s authorization prompt, where they can review and approve the requested permissions. The URL must include your app’s unique client ID, the scopes your app is requesting, and a redirect URI to which Shopify will send the access code upon consent. Constructing this URL correctly is vital for ensuring the store owner is presented with the correct information and that Shopify can redirect back to your app with the necessary authorization code.
Example: PHP Integration
Implementing the consent request process in PHP involves creating a script that generates the installation URL dynamically based on the store’s information and your app’s credentials. This script typically uses the store’s domain and your app’s client ID, scopes, and redirect URI to construct the URL. Once the URL is generated, you can redirect the store owner to this URL, initiating the consent process. This PHP script acts as the bridge between your app and Shopify, facilitating the authorization workflow.
Step Three: Securing the Access Code
Handling the Callback
Once the store owner grants consent, Shopify redirects them to your app’s redirect URI with an access code included in the request. This access code is temporary and must be exchanged for a permanent access token. The callback handler in your app needs to capture this code, validate it, and then use it in a subsequent request to Shopify’s token endpoint to obtain the access token. Properly handling this callback is crucial for the security and functionality of your app, as it transitions from the authorization phase to having authenticated access to the store’s data.
Verifying Information
Verifying the integrity and authenticity of the information received from Shopify, especially the access code, is a critical sub-step. This may involve validating the state parameter if used during the initial request to prevent cross-site request forgery (CSRF) attacks and ensuring the access code has not been tampered with. Implementing these verification steps helps secure the communication between your app, the store, and Shopify, maintaining the trust of all parties involved.
Illustration: PHP Implementation
A practical PHP code example for this step would involve setting up a listener at your redirect URI endpoint that can handle GET requests from Shopify. This script would extract the access code from the query parameters, validate it, and then use it to make a POST request to Shopify’s token endpoint. This request would include your app’s client ID and client secret to authenticate the request and the access code to be exchanged for an access token. Handling the response from Shopify correctly, extracting the access token, and storing it securely for future API requests is paramount for the app’s operation within the store.
Fourth Step: Transforming the Access Code into a Store Token
Making the API Call: Detailed Instructions for Exchanging the Access Code for a Permanent Access Token
After receiving the access code, the next critical step is to transform this code into a permanent access token. This process involves making a POST request to Shopify’s API. The request must include the app’s API key, the app’s API secret, the access code received from the previous step, and the specified redirect_uri used during the consent process. The endpoint for this request typically follows the pattern: https://{shop}.myshopify.com/admin/oauth/access_token
.
The body of the POST request should contain the necessary parameters encoded in JSON or form-urlencoded format, specifying the ‘client_id’ (API key), ‘client_secret’ (API secret), and ‘code’ (the access code). It’s crucial to ensure that the request is made securely and that the API key and secret are kept confidential to prevent unauthorized access.
Example: PHP Application: PHP Code Snippet for This Critical Step
A PHP example for this step might look something like the following:
<?php
$apiUrl = "https://{shop}.myshopify.com/admin/oauth/access_token";
$data = array(
'client_id' => 'your_api_key',
'client_secret' => 'your_api_secret',
'code' => 'the_received_access_code'
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($data));
$response = curl_exec($ch);
curl_close($ch);
$accessToken = json_decode($response)->access_token;
?>
This snippet sends a POST request to Shopify’s OAuth endpoint to exchange the access code for an access token, which is then parsed from the JSON response.
Fifth Phase: Executing API Requests
Preparing Your First API Call: Guidelines for Using the Access Token to Make Authenticated API Requests
With the access token obtained, you can now make authenticated API requests to Shopify. The access token should be included in the HTTP header of your requests as a Bearer token. It’s important to ensure that each request is made securely and that the access token is not exposed in any insecure contexts.
Sample: PHP Use Case: Example of Executing an API Request in PHP
Here’s a simple PHP example demonstrating how to make an API request to retrieve information about the shop:
<?php
$apiUrl = "https://{shop}.myshopify.com/admin/api/2021-04/shop.json";
$headers = array(
'Content-Type: application/json',
'Authorization: Bearer ' . $accessToken
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $apiUrl);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$shopInfo = json_decode($response);
?>
This code fetches details about the Shopify store using the access token for authentication.
Dynamic API Request Execution: Tips for Dynamically Constructing API Requests Based on Different Needs
When developing a Shopify app, you may need to execute different types of API requests based on various needs, such as fetching product details, updating inventory, or processing orders. Constructing these requests dynamically involves understanding the Shopify API endpoints and the required parameters for each type of request. Utilizing variables for parameters such as shop URL, API version, and resource identifiers can make your code more flexible and reusable.
The Value of Repetition: Understanding the Importance of Iterative Testing and Debugging in API Request Execution
The development of robust Shopify apps requires iterative testing and debugging, especially when executing API requests. This process helps identify any issues with request parameters, authentication, or handling of responses. Implementing logging and error handling mechanisms can aid in tracking down and resolving issues more efficiently. Regular testing across different scenarios ensures that your app behaves as expected and can handle errors gracefully, providing a reliable and user-friendly experience for Shopify merchants.
Generating Access Tokens via Shopify’s Custom Apps
Generating access tokens for Shopify’s custom apps involves a series of steps designed to ensure secure and authorized access to Shopify’s API. These tokens are crucial for custom apps to interact with Shopify stores programmatically, enabling a wide range of functionalities tailored to the specific needs of the store.
Key Steps for Success
When custom apps integrated with Shopify, the process of generating access tokens is pivotal. This process ensures that the app has the necessary permissions to perform its intended functions without compromising the security of the store’s data. It involves creating the app within the Shopify ecosystem, installing it on a target store, and then using the app’s credentials to request access tokens. These tokens are what the app uses to make authenticated calls to the Shopify API, acting as a key to unlock the API’s functionalities.
Initial Step: App Creation and Installation
The journey begins with the development of the custom app, which is tailored to address specific requirements that cannot be met by existing apps in the Shopify App Store. This custom solution involves selecting the appropriate scopes for API access, which determines what data and actions the app can perform on the Shopify store. Once the app is developed, the next step is to install it on the Shopify store. This installation process typically involves generating an installation link or using the Shopify admin to add the app directly to the store, depending on whether the app is intended for a single store or multiple stores. The store owner’s approval is necessary to complete the installation, granting the app the requested permissions.
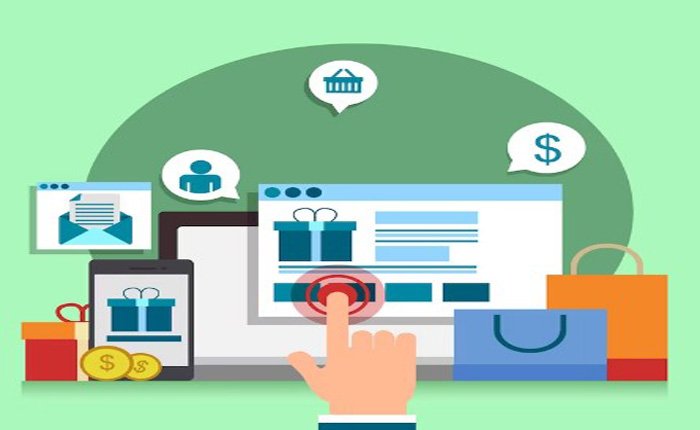
Second Step: Executing Authenticated Calls
Detailed Procedurese
With the app installed and the necessary permissions granted, the focus shifts to utilizing the access token. This token, obtained during the installation process, is used to authenticate API calls made by the app to the Shopify store. The access token ensures that these calls are securely authenticated, allowing the app to access and manipulate store data within the bounds of the permissions granted. This step is crucial for the app’s operation, as it involves sending and receiving data to and from the Shopify API, executing the app’s core functionalities.
Updating API Credentials for Apps Created by Admins
For apps that are created and managed by store admins, maintaining the security of API keys and secrets is paramount. These credentials are the foundation of the app’s secure interaction with Shopify’s API. Best practices for managing these credentials include regularly updating them to prevent unauthorized access and ensuring they are stored securely, away from potential threats. Additionally, limiting the distribution of these credentials and monitoring their use can help safeguard against security breaches. This practice not only protects the app and the store it operates in but also maintains the integrity of the data being handled.
Important Considerations
When developing and managing Shopify custom apps, there are crucial considerations to ensure the app functions effectively and securely within the Shopify ecosystem. Two of the most significant considerations are modifying API scopes and understanding the necessary permissions for allocating those scopes to custom apps.
Modifying API Scopes
API scopes define the extent of access that an app has to a Shopify store’s data. When developing a custom app, selecting the appropriate API scopes is crucial for ensuring the app can perform its intended functions without overreaching into unnecessary data areas, which could raise security and privacy concerns. As the needs of the app or the store evolve, you may find it necessary to adjust these scopes to either increase the app’s capabilities or restrict its access to sensitive data.
Adjusting your app’s API scopes involves understanding the specific requirements of your app’s functionality and the data it needs to access. If you need to expand your app’s functionality, you might need to request additional permissions from the store owner. This process typically requires generating a new installation or permission URL with the updated scopes and having the store owner approve these changes. It’s essential to communicate clearly with the store owner about why these changes are necessary and how they will impact the app’s operation and the store’s data security.
Necessary Permissions for Allocating Scopes to Custom Apps
Allocating scopes to custom apps requires a clear understanding of the permissions system within Shopify. Permissions to assign or modify scopes come from the level of access the app developer or the store admin has within the Shopify environment. For a custom app, the initial set of scopes is usually defined during the app development process, with the consent of the store owner being a critical step in granting those scopes.
Store owners must explicitly agree to the scopes requested by the app, highlighting the importance of transparency in communicating what data the app will access and for what purposes. The Shopify admin interface provides mechanisms for managing these permissions, allowing store owners to review and control the level of access granted to each custom app installed on their store. Understanding these permissions and how to navigate them is crucial for both developers and store owners to maintain the security and functionality of the store while leveraging the benefits of custom apps.
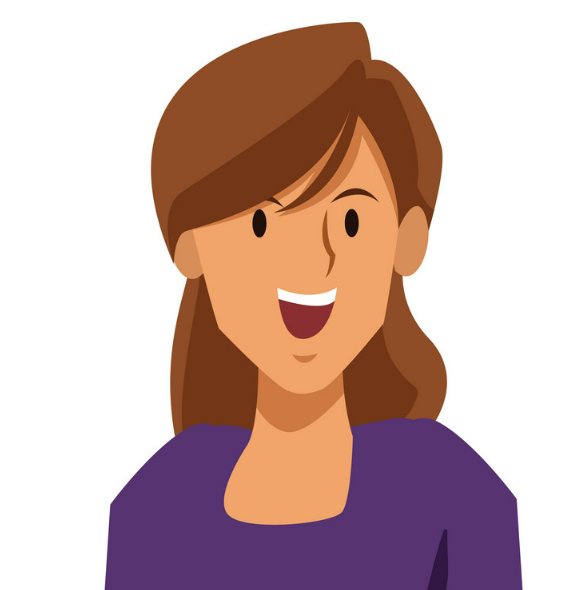
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.