Introduction to Python’s Web Connectivity with urllib
Python, with its comprehensive standard library, offers robust capabilities for interacting with the internet, making web operations like data fetching, API calls, and website scraping straightforward for developers. Among the toolkit provided by Python for web connectivity, the urllib
module stands out as a versatile and easy-to-use tool.
Urllib Module as a Tool for Web Operations
The urllib
module in Python is designed for handling URL operations. It is a package that bundles several submodules for various web-related tasks, including fetching data across the web, parsing URLs, and handling HTTP requests and responses. This module abstracts the complexities of HTTP communication, making it more accessible to developers.
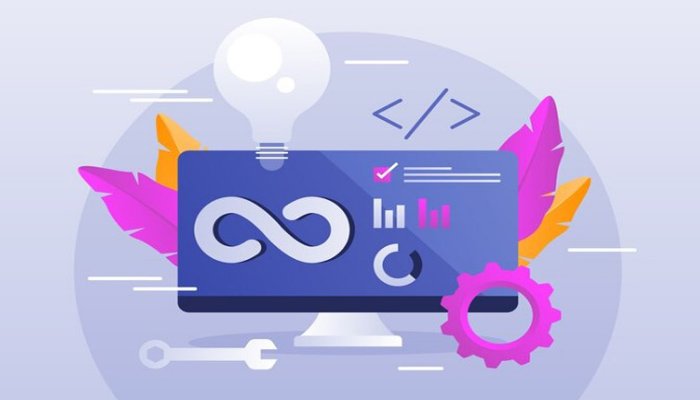
Getting Started with urllib: The Basics
The urllib
module encapsulates a range of functionalities necessary for web programming, from sending requests to processing the responses received from web servers.
Understanding the urllib Module and Its Significance
urllib
is significant in the Python ecosystem for its comprehensive approach to web connectivity. It comprises several components: request
for opening and reading URLs, response
for handling the response from requests, error
for managing exceptions and errors, parse
for parsing URLs, and robotparser
for parsing robots.txt
files, thus ensuring compliance with website policies.
Making Your First URL Request Using urllib.request
The urllib.request
submodule allows for fetching URLs. A basic usage involves importing the submodule and using the urlopen
method to fetch the content of a webpage. The method returns a response object from which data can be read.
Practical Examples of Simple Web Requests
A simple example to fetch and print the content of a webpage would involve:
import urllib.request
with urllib.request.urlopen('http://example.com') as response:
html = response.read()
print(html)
This code snippet demonstrates how to open a URL and read its contents, printing the HTML of the specified webpage.
Navigating Web URLs with Python: A Closer Look at urllib.request
urllib.request
offers more than just fetching URLs; it provides a detailed interface for managing web requests.
Detailed Explanation of urllib.request Functions
The urllib.request
submodule includes functions for adding headers to requests, handling authentication, and managing cookies, among others. For example, using Request
objects to set HTTP headers allows for simulating browser requests or making API calls that require specific headers.
How to Handle HTTP Response Headers and Metadata
Handling response headers involves using the .info()
method on the response object, which provides access to headers such as content type, server, and date. This metadata can be crucial for tasks like content negotiation or caching strategies.
Practical Guide to Web Requests: From Code to Response
Executing web requests in Python using urllib
is straightforward but requires attention to detail, especially when handling more complex scenarios like form submissions or dealing with redirects.
Step-by-Step Guide on Executing Web Requests in Python Code
To execute a web request, one typically starts by constructing a Request
object with any necessary headers, then uses urlopen
to fetch the response. Handling exceptions is crucial, as web requests can fail due to network issues, server errors, or incorrect URLs.
Examples Showcasing Typical Use Cases and Handling Exceptions
A typical use case might involve submitting a form using urllib
. This would require encoding the form data and adding it to the request. Exception handling would involve catching URLError
or HTTPError
exceptions to gracefully handle errors.
Decoding Web Responses: How to Interpret Data
Once a web request is made and a response is received, the next step is interpreting the data.
Techniques for Reading and Decoding the Response from a Web Request
The response data can be read using the .read()
method, and if the data is binary, it may need to be decoded to a string format using the appropriate character encoding, typically UTF-8.
Handling Different Data Formats and Character Encodings
Web responses can be in various formats, including JSON, HTML, or plain text. Handling these formats involves decoding the response body and, in the case of JSON, using the json
module to parse the string into a Python dictionary for easier manipulation. Handling character encodings correctly ensures that the data is accurately represented when processed or displayed.
Advanced urllib Techniques: Beyond Basic URL Fetching
Exploring the depths of urllib
reveals its potential not just for fetching URLs, but for a broad spectrum of web interaction tasks, including web scraping and data extraction.
Reading and Parsing HTML Content from URLs
Once a web page’s content is fetched using urllib
, parsing the HTML to extract specific information is often the next step. Libraries such as BeautifulSoup or lxml can be utilized in tandem with urllib
to navigate the HTML DOM tree and extract data, such as headlines, paragraphs, and links, efficiently.
Techniques for Scraping Web Pages and Extracting Information
Web scraping with urllib
involves more sophisticated techniques for interacting with web pages. This can include handling cookies to maintain session information, using regular expressions or XPath queries to pinpoint data within the HTML structure, and managing pagination to scrape data across multiple pages.
Compatibility Considerations: urllib in Python 2 vs Python 3
The transition from Python 2 to Python 3 brought significant changes to the urllib
module, affecting how developers write scripts for web interactions.
Differences in urllib Usage between Python Versions
In Python 2, urllib
, urllib2
, and urlparse
were separate modules, each handling different aspects of URL actions. Python 3 unified these functionalities under urllib
, dividing them into submodules like urllib.request
, urllib.parse
, and urllib.error
. This change streamlined web operations but required a shift in how URLs are requested and processed.
Tips for Ensuring Backward Compatibility with Python 2
For projects needing to support both Python 2 and 3, using a compatibility layer like six
or future
can help bridge the differences in urllib
usage. Alternatively, conditional imports based on the Python version can maintain compatibility, though this approach may complicate the codebase.
Mastering urllib: Tips, Tricks, and Best Practices
Efficient and safe web requests are pivotal in web scraping and data extraction projects. Mastering urllib
involves adopting best practices and being aware of common pitfalls.
Use Headers
Mimicking a real browser by incorporating headers in your requests can significantly reduce the chances of your bot being detected and subsequently blocked by websites. This technique involves setting user-agent strings and other headers that emulate browser requests, making your script’s requests appear legitimate to web servers.
Manage Sessions with http.cookiejar
For websites that necessitate login to access certain content, managing session cookies is vital. The http.cookiejar
module in Python can be used to store and transmit cookies during your session, thereby maintaining the state of your login across multiple requests to the same site.
Rate Limiting
Incorporating delays between your requests is a respectful practice that helps prevent overwhelming the server, which can lead to your IP address being banned. Implementing rate limiting can be as simple as using the time.sleep()
function in Python to add a pause between requests.
Error Handling
Utilizing try-except blocks allows your script to gracefully handle exceptions that may occur during web requests, such as network issues, HTTP errors, or problems parsing URLs. This practice ensures that your script can recover from errors or at least fail gracefully, providing debug information for troubleshooting.
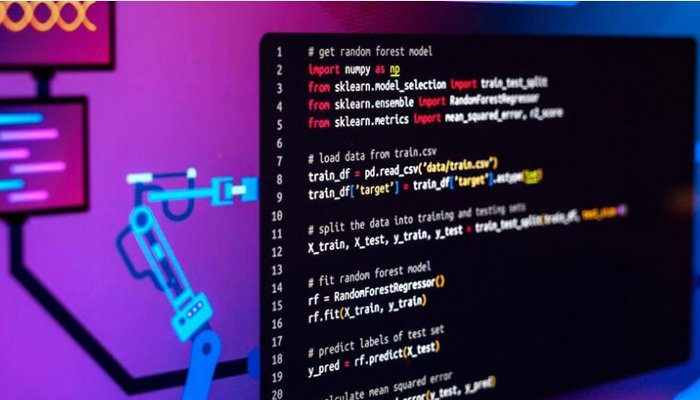
Common Pitfalls to Avoid and How to Troubleshoot Common Issues
Ignoring Robots.txt
Before scraping a website, it’s crucial to review its robots.txt
file to ensure that your scraping activities are permitted. This file outlines which parts of the site are off-limits to bots, helping you avoid legal issues and respect the website’s guidelines.
Hardcoding URLs
Hardcoded URLs can become a point of failure in your script, especially with dynamic websites where URLs may change. Utilizing the urllib.parse
module to dynamically construct URLs ensures that your script remains functional even if the website’s URL schema changes.
Not Checking the MIME Type
Verifying the MIME type of the content you’re downloading is essential to ensure it matches your expectations. This can be done by checking the Content-Type
header in the HTTP response, helping you avoid processing unwanted content types.
Debugging
Effective debugging involves using verbose output or logging to track the request-response cycle closely. This can help identify issues with your requests or understand how the server responds to them. Additionally, network debugging tools like Wireshark or the developer tools in web browsers can provide valuable insights into the HTTP communication process.
Practical Applications of urllib in Real-World Projects
The versatility of urllib
extends its utility beyond simple web requests, enabling its integration into complex, real-world applications. From web scraping to API interactions, urllib
serves as a foundational tool that, when combined with other Python libraries, unlocks a vast potential for automating and enhancing internet-related tasks.
Web Scraping with urllib
urllib
is frequently utilized for downloading web pages, which are subsequently parsed for information using libraries such as BeautifulSoup or lxml. This method is particularly effective in projects that compile data from various sources, like price comparison websites or news aggregators, where the objective is to gather vast amounts of data from the internet efficiently.
API Interactions Using urllib
RESTful APIs, which return data in JSON or XML format, are commonly accessed using urllib
for making requests. This data can then be processed with Python’s built-in json
or xml
modules, catering to a wide range of applications from social media analytics to automated reporting systems. This showcases urllib
‘s capability in facilitating interactions with web services and processing their responses for further analysis or display.
Dynamic Content Retrieval with urllib
For websites that dynamically load content using JavaScript, urllib
can be employed to fetch the initial HTML. Subsequent HTTP requests can retrieve the dynamic content, often requiring parsing with libraries like json
. This technique is crucial for scraping modern web applications that rely heavily on AJAX calls to load data.
Integrating urllib with Data Processing Libraries
Once data is fetched via urllib
, libraries like Pandas can be leveraged for further data analysis and visualization. This integration allows for the sophisticated processing of web-scraped data, transforming raw data into insightful information suitable for decision-making or reporting.
Enhancing urllib with Asynchronous Requests
In scenarios demanding high-performance web scraping or API interactions, urllib
can be integrated with asyncio
to enable asynchronous requests. This combination significantly improves the speed and efficiency of web operations, catering to projects with extensive data collection needs.
Leveraging urllib in Machine Learning Projects
Data collected through urllib
, either via web scraping or API interactions, can be instrumental in feeding machine learning models. Libraries such as scikit-learn or TensorFlow can utilize this data for various analyses, including predictive modeling and sentiment analysis, underscoring urllib
‘s role in the data collection phase of AI projects.
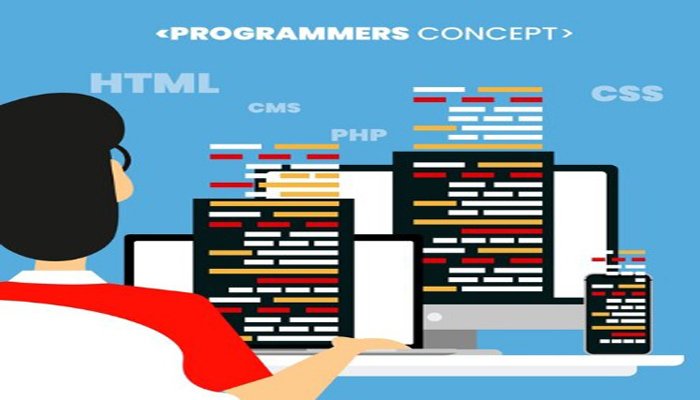
Conclusion: Harnessing the Power of Python’s urllib for Internet Access
Throughout this exploration of Python’s urllib
, we’ve uncovered the module’s extensive capabilities for web connectivity, from basic URL fetching to advanced web scraping and API interactions. The integration of urllib
with other powerful Python libraries further extends its utility, enabling developers to tackle a wide array of projects that require internet access.
Recap of the Key Points Covered
We’ve delved into the basics of urllib
, covering its components like request
, response
, and error
, and provided practical examples of making web requests and handling responses. We’ve also explored advanced techniques for web scraping, discussed compatibility considerations between Python versions, and highlighted best practices for efficient and secure web operations.
Encouragement to Experiment and Explore urllib’s Potential
The journey through urllib
and its applications is just the beginning. As you become more familiar with its functionality, you’re encouraged to experiment with its features, integrate it with other libraries, and explore its potential in your projects. Whether for data collection, API interactions, or web scraping, urllib
offers a solid foundation for accessing and interacting with the web in Python. The versatility and power of urllib
, coupled with the Python ecosystem, provide an unparalleled toolkit for developers looking to harness the full potential of internet connectivity in their projects.
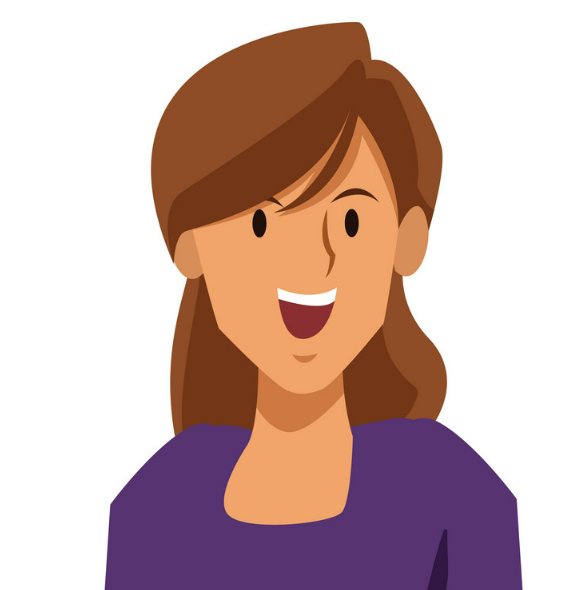
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.