Introduction
The integration of MySQL with PHP represents a cornerstone in web development, offering a powerful combination for creating dynamic, data-driven websites. MySQL, a robust database management system, is known for its reliability, speed, and ease of use. On the other hand, PHP is a server-side scripting language designed specifically for web development, providing flexibility and simplicity in coding. The synergy between MySQL and PHP enables developers to build scalable, secure, and efficient web applications, making it a preferred choice for many web development projects.
Getting Started with MySQL
An Introduction to MySQL: What You Need to Know
MySQL is an open-source relational database management system (RDBMS) that uses Structured Query Language (SQL) for database access. It is designed to handle large volumes of data, providing an efficient way to store, modify, and retrieve data. MySQL is widely recognized for its performance and reliability, making it an essential tool for web developers.
Basic Concepts of MySQL
MySQL operates on a client-server model, where the server runs on a machine hosting the database, and clients connect to the server to access the databases. It supports various data types, including numeric, date and time, and string types, allowing for a versatile data storage mechanism. The fundamental concepts of tables, rows, and columns form the basis of MySQL’s data organization, with SQL commands used to perform operations such as data insertion, updates, deletions, and queries.
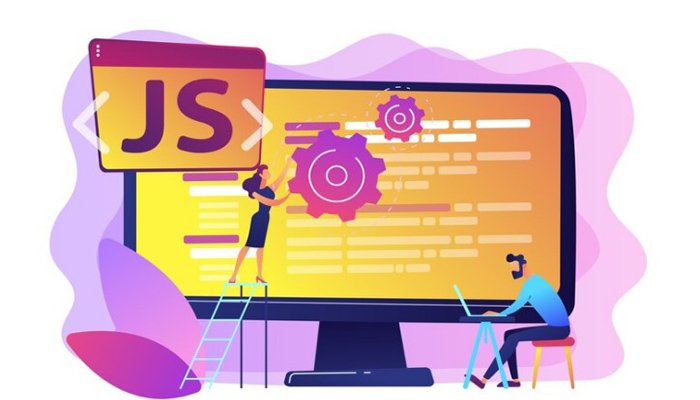
Why MySQL is Preferred for Web Projects
MySQL’s popularity in web projects stems from its compatibility with all major hosting providers, ease of integration with PHP and other programming languages, and its open-source nature, which makes it cost-effective for developers. Its performance, backed by features like indexing and transaction support, ensures that web applications run smoothly and efficiently.
The Advantages of Using MySQL for Your Projects
The performance, reliability, and scalability of MySQL are key benefits that make it an attractive option for web projects. It can handle a significant amount of data without compromising on speed, making it suitable for websites with high traffic volumes. Additionally, MySQL’s advanced security features, including encrypted connections and password protection, ensure data safety.
MySQL Versus MariaDB: A Detailed Comparison
Comparing MySQL and MariaDB reveals both similarities and differences. MariaDB, a fork of MySQL, maintains compatibility with MySQL but also introduces new features and storage engines. Both systems offer high performance, comprehensive security measures, and are ACID-compliant, ensuring reliable transaction processing. However, MariaDB is often praised for its open-source commitment and the inclusion of cutting-edge features, which may offer additional benefits over MySQL in certain scenarios. The choice between MySQL and MariaDB ultimately depends on specific project requirements and developer preference.
Essential System Requirements for Running MySQL
Running MySQL efficiently requires meeting certain hardware and software prerequisites. The system requirements vary based on the workload, but generally, a server with adequate memory, a multi-core CPU, and sufficient disk space is recommended. On the software side, MySQL supports a wide range of operating systems, including Linux, Windows, and macOS, ensuring flexibility in different development environments.
Setting Up MySQL and PHP
The MySQLi (“MySQL Improved”) extension provides a way for PHP scripts to interact with MySQL databases. It offers both procedural and object-oriented programming interfaces, giving developers the flexibility to choose the best approach for their project. The MySQLi extension includes features such as prepared statements, which enhance security by preventing SQL injection attacks, and transaction support, which ensures data integrity. The integration of MySQLi in PHP projects significantly improves performance, offers better error handling capabilities, and ensures a more secure database interaction, making it a crucial component in modern web development.
Leveraging PHP Data Objects (PDO) for Database Interactions
PHP Data Objects (PDO) represent a database access layer providing a uniform method of access to multiple databases. Unlike the MySQLi extension, which works only with MySQL databases, PDO supports over twelve different database drivers, offering a significant advantage in terms of database flexibility and portability of code. PDO also provides a consistent API for database operations, making it easier for developers to switch between database systems without needing to rewrite SQL queries or connection scripts. Additionally, PDO offers robust security features, such as prepared statements, that protect against SQL injection attacks. Its object-oriented approach simplifies the process of database interaction, making code cleaner and more maintainable.
Setting Up Your MySQL Database Locally
Step-by-step Guide to Installing and Configuring MySQL on a Local Machine
Setting up MySQL locally involves downloading the MySQL server from the official website and following the installation instructions specific to your operating system. During installation, you’ll be prompted to configure settings such as the root password, which is crucial for securing your database. Post-installation, you can access the MySQL server through the command line or use a graphical interface like MySQL Workbench to manage your databases more visually. The configuration process may include setting up paths, configuring network options, and creating a dedicated MySQL user for better security.
Initial Database Creation Steps
Creating your first database is a fundamental step in starting your web project. This process can be initiated using the MySQL command line or a graphical user interface, where you execute a CREATE DATABASE
SQL statement followed by the database name. Understanding basic operations such as creating tables (CREATE TABLE
), inserting data (INSERT INTO
), updating records (UPDATE
), and querying data (SELECT
) is crucial at this stage. These operations form the backbone of database interaction within your web applications.
Organizing Your Project: Structuring Folders in htdocs
Best practices for organizing your web project files involve creating a clear and logical directory structure within the htdocs
folder (or the equivalent in your web server environment). This structure typically includes separate folders for scripts (php
), stylesheets (css
), JavaScript files (js
), and images (img
). Such organization ensures that your project is maintainable and scalable, making it easier to manage as it grows in complexity.
Connecting PHP to MySQL
Crafting the PHP Database Connection Script
Writing a PHP script to connect to a MySQL database involves using either the MySQLi extension or PDO. The script includes specifying your database host, username, password, and the database name you wish to connect to. With PDO, the connection string also specifies the database type. This script typically initializes a new instance of the MySQLi or PDO class, handling any exceptions or errors to ensure a secure connection is established.
Verifying Your Database Connection
Ensuring your PHP script successfully connects to MySQL involves checking the connection for errors. With MySQLi, this could mean checking if the connection object’s connect_error
property is set. For PDO, you would catch any exceptions thrown during the creation of the PDO object. Handling these errors properly allows for debugging and ensures that your application can gracefully handle connection issues.
Confirming Connection Success with a Message
Implementing user feedback for successful database connections can significantly enhance the user experience. This typically involves displaying a confirmation message upon a successful connection. In a development environment, this could be as simple as echoing a “Connection successful” message in PHP. For production environments, however, it’s crucial to log success without exposing sensitive information or connection details, adhering to best practices for security and user experience.
Advanced Integration Techniques
Implementing MySQL Database on Cloudways
Using Cloudways for managing MySQL databases simplifies the deployment and management of web applications on cloud infrastructure. Cloudways offers a managed cloud hosting platform that allows developers to deploy, manage, and scale web applications with ease. The platform supports various cloud providers, including AWS, Google Cloud, and DigitalOcean, providing a flexible environment for MySQL database hosting. Through its intuitive dashboard, developers can launch a managed MySQL server, adjust server settings, and manage database backups with just a few clicks. Cloudways also emphasizes security and performance, offering features such as automated backups, SSL certification, and dedicated firewalls.
Procedure-Oriented MySQLi Connection vs. Utilizing PDO
Comparing procedural MySQLi and PDO for database connections highlights the flexibility and security offered by PDO. While MySQLi provides both procedural and object-oriented interfaces, it is limited to MySQL databases. Procedural MySQLi is simpler and more straightforward, making it suitable for beginners or small projects. On the other hand, PDO supports multiple database types and offers a consistent object-oriented approach. PDO’s prepared statements enhance security against SQL injection attacks, and its ability to handle exceptions makes error management more robust. The choice between MySQLi and PDO depends on the project’s requirements, developer preference, and the need for database flexibility.
Configuring Remote MySQL Access
Enabling and securing MySQL access over the internet is crucial for remote database management and application integration. To configure remote access, you must adjust the MySQL server’s settings to listen for connections from outside the localhost, typically by modifying the my.cnf
or my.ini
file. It’s also necessary to create or modify user privileges to allow access from specific or all IP addresses. Security measures, such as SSL encryption and VPN tunnels, are essential to protect data in transit. Additionally, using firewalls to restrict access to the MySQL port (default is 3306) to known IPs can significantly enhance security.
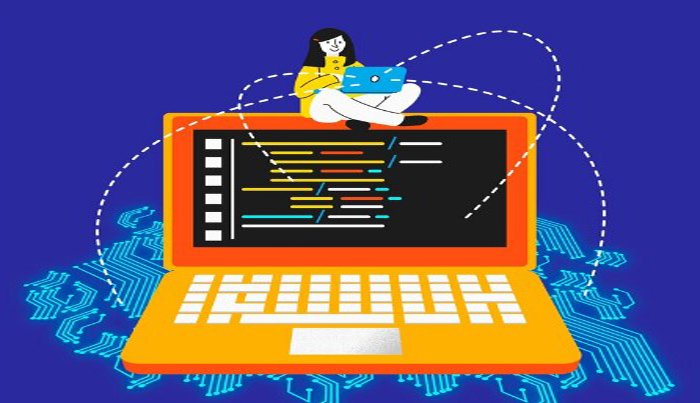
Tools and Optimization
Top Tools for MySQL Management and Efficiency
MySQL Workbench, Navicat, and SQLyog stand out as top tools for MySQL management and efficiency. MySQL Workbench, an official Oracle tool, provides a comprehensive environment for database design, administration, and development. Navicat offers a user-friendly interface and powerful features for database management, including synchronization, backup, and data transfer between databases. SQLyog is known for its simplicity and efficiency, providing a straightforward GUI for database administration, with features like schema comparison, query builder, and scheduled backups. These tools cater to different preferences and requirements, enhancing productivity and management capabilities for MySQL databases.
Elevating Database Efficiency Through Cloudways Optimization
Optimizing MySQL databases on cloud platforms like Cloudways involves leveraging the platform’s features and best practices to enhance performance. Cloudways provides optimized stack configurations, including advanced caching mechanisms and a dedicated environment for MySQL. Regularly monitoring database performance through Cloudways’ platform allows for identifying and addressing bottlenecks. Implementing indexing, optimizing queries, and ensuring efficient use of resources can further improve database efficiency. Cloudways also supports vertical scaling, enabling easy adjustment of server resources to meet the demands of growing applications.
Practical Implementation
Connecting HTML to MySQL Database Via PHP: A Practical Example
Linking a simple HTML form to a MySQL database via PHP involves creating an HTML form that collects user input, and a PHP script to process the form data and store it in the database. The process starts with designing an HTML form with fields for the data to be collected. The form’s action attribute is set to point to the PHP script responsible for handling the submission. The PHP script then uses either MySQLi or PDO to connect to the database, validate the form data, and execute an INSERT SQL statement to add the data to the database. Error handling is crucial to manage any exceptions or issues that arise during the process.
Essential Tools for Linking HTML Forms to MySQL Database with PHP
Tools and libraries that facilitate form to database connectivity enhance the development process, offering validation, security, and database interaction features. Libraries such as jQuery and Axios can be used to submit form data asynchronously, improving user experience. For server-side processing, PHP frameworks like Laravel provide built-in functionalities for validation, ORM (Object-Relational Mapping) for database interactions, and security against common vulnerabilities. These tools streamline the development workflow, ensuring that data is efficiently and securely processed and stored in the MySQL database.
Preparing Your HTML Contact Form: A Prerequisite Analysis
Before diving into the creation of an HTML contact form, it’s crucial to analyze the design considerations and preparation needed. The form should be intuitive and user-friendly, ensuring users can easily enter their information. Consider the types of data you intend to collect, such as name, email, and message, and how these data points align with the database structure you plan to use. Also, think about the user experience, including form validation feedback and responsive design to accommodate various devices. Preparing for these aspects in advance lays the groundwork for a seamless integration with MySQL.
Database and Table Creation in MySQL: Laying the Foundations
Creating databases and tables in MySQL is the first technical step in preparing for form data storage. Start by accessing your MySQL server through a command line or a management tool like MySQL Workbench. Use the CREATE DATABASE
statement to create a new database for your form data. Following that, create a table within this database using the CREATE TABLE
command, defining columns that correspond to the data you’ll collect from your HTML form, such as id
, name
, email
, and message
. Specify appropriate data types for each column to ensure data integrity and efficient storage.
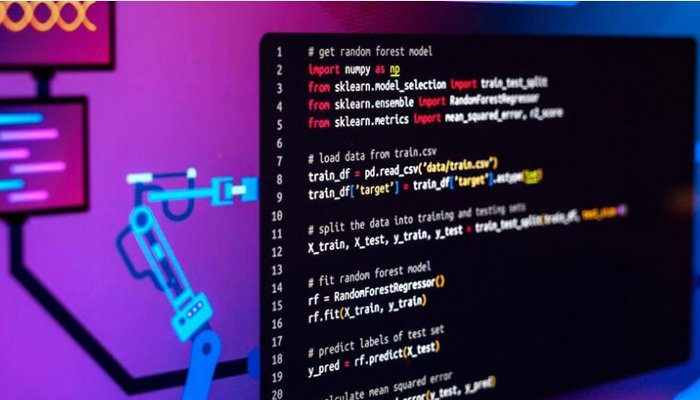
Designing an HTML Form for Database Connectivity
When designing an HTML form for database connectivity, include key elements that facilitate effective MySQL integration. Each input field should have a name
attribute that matches the column names in your MySQL table, ensuring data mapping is straightforward during the processing stage. Include form fields for all the data you wish to collect and consider using HTML5 validation attributes to improve user input. Additionally, a clear submit button is essential. For enhanced user interaction, you might also integrate JavaScript or AJAX to submit the form data without needing to reload the page.
Developing a PHP Script to Store HTML Form Data in MySQL
The PHP backend plays a crucial role in processing and storing form submissions in the MySQL database. Start by establishing a connection to your MySQL database using PDO or MySQLi, ensuring to handle any potential errors during the connection process. Once connected, prepare your SQL query to insert the form data into the database, using prepared statements to prevent SQL injection attacks. Sanitize and validate the incoming data to ensure it meets your requirements before it’s stored. After executing the insert operation, provide feedback to the user, such as a confirmation message or error notification if the process fails.
Completion and Testing: Verifying the Setup
Testing your integration is critical to ensure proper functionality. Begin with unit tests to verify that each component of your setup works as expected in isolation—test the HTML form’s user interface, the PHP backend’s ability to process and store data, and the MySQL database’s structure and data integrity. Following unit testing, conduct end-to-end tests to simulate the user’s experience and confirm that the form submission process works seamlessly from start to finish. Pay attention to error handling and user feedback during these tests to refine the user experience.
Conclusion
Recap of MySQL and PHP Integration Essentials
The integration of MySQL and PHP for handling HTML form submissions encompasses several key components: designing a user-friendly form, setting up a MySQL database and tables, crafting a PHP script to process form data, and thoroughly testing the setup. Each step requires careful consideration and execution to ensure data is captured, stored, and managed effectively.
Final Thoughts on Optimizing Your Web Development Workflow with MySQL and PHP Integration
Optimizing your web development workflow with MySQL and PHP integration involves embracing best practices such as using prepared statements for database security, implementing client-side and server-side validation for data integrity, and conducting thorough testing to ensure reliability. Additionally, staying informed about the latest features and updates in PHP and MySQL can help enhance your applications’ efficiency and security. By carefully planning your form, database structure, and backend processing script, you can create a seamless and secure system for managing form submissions, thereby enhancing the overall user experience and reliability of your web applications.
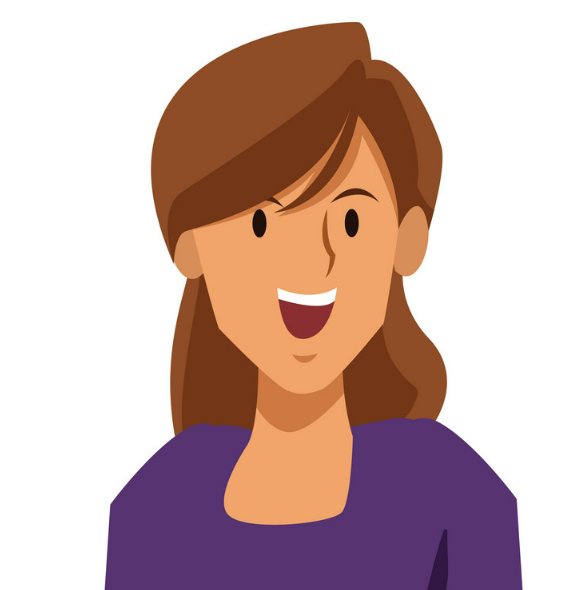
As a seasoned professional with a unique blend of skills in Computer Design and Digital Marketing, I bring a comprehensive perspective to the digital landscape. Holding degrees in both Computer Science and Marketing, I excel in creating visually appealing and user-friendly designs while strategically promoting them in the digital world.